Keyboard
The keyboard module provides methods for retrieving input from the keyboard.
Detecting Key Presses
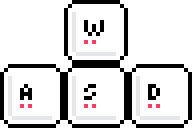
You can check if a key is currently pressed (and held down) using the isDown
method. This method takes a single argument, which is the key to check specified as a string.
function update() {
if (keyboard.isDown('w')) {
// Move the player up
}
if (keyboard.isDown('a')) {
// Move the player left
}
if (keyboard.isDown('s')) {
// Move the player down
}
if (keyboard.isDown('d')) {
// Move the player right
}
}
To check if a key was pressed this frame, use the wasPressed
method. This method takes a single argument, which is the key to check specified as a string.
function update() {
if (keyboard.wasPressed('i')) {
// Open the inventory
}
}
The difference between isDown
and wasPressed
is that isDown
will return true
as long as the key is held down, while wasPressed
will only return true
once per key press.
Detecting Key Releases
You can check if a key is currently released (and not held down) using the isUp
method. This method takes a single argument, which is the key to check specified as a string.
function update() {
if (keyboard.isUp('space')) {
// Space bar is not pressed
}
}
To check if a key was released this frame, use the wasReleased
method. This method takes a single argument, which is the key to check specified as a string.
function update() {
if (keyboard.wasReleased('space')) {
// Space bar was released this frame
}
}
Similar to isDown
and wasPressed
, the difference between isUp
and wasReleased
is that isUp
will return true
as long as the key is not held down, while wasReleased
will only return true
once per key release.
Key Codes
The following table lists the key codes for each key on the keyboard.
Key Code | Key | Scan Code | Unicode |
---|---|---|---|
3 | Cancel | cancel | |
8 | Backspace | backspace | ⌫ |
9 | Tab | tab | ↹ |
12 | Clear | clear | ⌧ |
13 | Enter | enter | ⏎ |
16 | Shift | shiftleft | ⇧ |
16 | Shift | shiftright | ⇧ |
17 | Control | controlleft | ⌃ |
17 | Control | controlright | ⌃ |
18 | Alt | altleft | ⌥ |
18 | Alt | altright | ⌥ |
19 | Pause | pause | ⎉ |
20 | Caps Lock | capslock | ⇪ |
27 | Escape | escape | ⎋ |
32 | Space | space | ␣ |
33 | Page Up | pageup | ⇞ |
34 | Page Down | pagedown | ⇟ |
35 | End | end | ⇟ |
36 | Home | home | ⇞ |
37 | Left Arrow | left | ← |
38 | Up Arrow | up | ↑ |
39 | Right Arrow | right | → |
40 | Down Arrow | down | ↓ |
42 | Print Screen | printscreen | |
45 | Insert | insert | ⌤ |
46 | Delete | delete | ⌦ |
48 | 0 | 0 | 0 |
49 | 1 | 1 | 1 |
50 | 2 | 2 | 2 |
51 | 3 | 3 | 3 |
52 | 4 | 4 | 4 |
53 | 5 | 5 | 5 |
54 | 6 | 6 | 6 |
55 | 7 | 7 | 7 |
56 | 8 | 8 | 8 |
57 | 9 | 9 | 9 |
65 | A | a | A |
66 | B | b | B |
67 | C | c | C |
68 | D | d | D |
69 | E | e | E |
70 | F | f | F |
71 | G | g | G |
72 | H | h | H |
73 | I | i | I |
74 | J | j | J |
75 | K | k | K |
76 | L | l | L |
77 | M | m | M |
78 | N | n | N |
79 | O | o | O |
80 | P | p | P |
81 | Q | q | Q |
82 | R | r | R |
83 | S | s | S |
84 | T | t | T |
85 | U | u | U |
86 | V | v | V |
87 | W | w | W |
88 | X | x | X |
89 | Y | y | Y |
90 | Z | z | Z |
91 | Left Meta | metaleft | ⌘ |
91 | Right Meta | metaright | ⌘ |
96 | Numpad 0 | numpad0 | 0 |
97 | Numpad 1 | numpad1 | 1 |
98 | Numpad 2 | numpad2 | 2 |
99 | Numpad 3 | numpad3 | 3 |
100 | Numpad 4 | numpad4 | 4 |
101 | Numpad 5 | numpad5 | 5 |
102 | Numpad 6 | numpad6 | 6 |
103 | Numpad 7 | numpad7 | 7 |
104 | Numpad 8 | numpad8 | 8 |
105 | Numpad 9 | numpad9 | 9 |
106 | Numpad * | numpadmultiply | * |
107 | Numpad + | numpadadd | + |
109 | Numpad - | numpadsubtract | - |
110 | Numpad . | numpaddecimal | . |
111 | Numpad / | numpaddivide | / |
112 | F1 | f1 | F1 |
113 | F2 | f2 | F2 |
114 | F3 | f3 | F3 |
115 | F4 | f4 | F4 |
116 | F5 | f5 | F5 |
117 | F6 | f6 | F6 |
118 | F7 | f7 | F7 |
119 | F8 | f8 | F8 |
120 | F9 | f9 | F9 |
121 | F10 | f10 | F10 |
122 | F11 | f11 | F11 |
123 | F12 | f12 | F12 |
144 | Num Lock | numlock | |
145 | Scroll Lock | scrolllock | |
160 | ^ | ^ | ^ |
161 | ! | ! | ! |